前端一键打包上传
大约 2 分钟
前端一键打包上传
目录结构
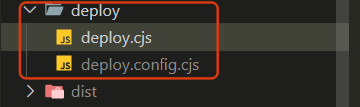
需要安装的包
{
"devDependencies": {
"archiver": "^5.3.1",
"node-ssh": "^13.2.0",
"ora": "^5.0.0",
"postcss-pxtorem": "^6.0.0"
}
}
具体代码
deploy.config.cjs
配置文件
let config = {
privateKey: '', // 本地私钥地址,位置一般在C:/Users/xxx/.ssh/id_rsa,非必填,有私钥则配置
passphrase: '', // 本地私钥密码,非必填,有私钥则配置
projectName: 'ZRAdmin', //项目名称
zipName: 'ZRAdmin.zip', //压缩包名称
host: '127.0.0.1', // 服务器ip
port: '22', // 端口一般默认22
username: 'root', // 用户名
password: '你的密码', // 密码
serverPath: '/usr/local/nginx/html/zradmin', // 上传到服务器的位置
distPath: './dist' // 本地打包文件的位置
};
module.exports = config;
deploy.cjs
const ora = require('ora');
const path = require('path');
const fs = require('fs');
const { NodeSSH } = require('node-ssh');
const ssh = new NodeSSH();
const chalk = require('chalk');
const archiver = require('archiver');
const projectDir = process.cwd();
console.log('============== 我是分割线 ==============');
console.log('项目路径', projectDir);
// 开始打包
function startZip(config) {
let { distPath, host } = config;
const tempPath = path.resolve(projectDir, distPath);
console.log('> 打包成zip');
const archive = archiver('zip', {
zlib: { level: 9 }
}).on('error', (err) => {
throw err;
});
const zipPath = path.join(projectDir, 'zip', config.zipName);
const output = fs.createWriteStream(zipPath).on('close', (err) => {
if (err) {
console.log(' 关闭archiver异常:', err);
return;
}
console.log(chalk.blue(' zip打包成功,' + zipPath));
console.log(`> 开始连接${chalk.underline.cyan(host)}`);
uploadFile(config, zipPath);
});
archive.pipe(output);
archive.directory(tempPath, '/');
archive.finalize();
}
// 上传文件
function uploadFile(config, zipPath) {
const { host, port, username, password, privateKey, passphrase } = config;
const sshConfig = {
host,
port,
username,
password
// privateKey,
// passphrase
};
ssh
.connect(sshConfig)
.then((res) => {
var remoteFilePath = `${config.serverPath}/${config.zipName}`;
console.log(chalk.blue(` SSH连接成功`));
console.log(`> 开始上传`);
const spinner = ora({ text: '正在上传中...', spinner: 'dots' });
spinner.color = 'blue';
spinner.start();
ssh
.putFile(zipPath, remoteFilePath)
.then(() => {
spinner.stop();
console.log(chalk.blue(` zip包成功上传至目录${chalk.underline.cyan(remoteFilePath)}`));
console.log('> 开始解压zip包');
statrRemoteShell(config);
})
.catch((err) => {
console.log(chalk.red(' 文件传输异常', err));
process.exit(0);
});
})
.catch((err) => {
console.log(chalk.red(' 连接失败', err));
process.exit(0);
});
}
// 开始执行远程命令
function statrRemoteShell(config) {
const { serverPath, projectName } = config;
const commands = [`cd ${serverPath}`, 'pwd', `unzip -o ${config.zipName}`];
const promises = [];
for (let i = 0; i < commands.length; i += 1) {
promises.push(runCommand(commands[i], serverPath));
}
Promise.all(promises)
.then(() => {
console.log(chalk.blue(' 解压成功'));
// console.log('(6)开始删除本地dist.zip')
// deleteLocalZip(config)
console.log(chalk.blue(`\n ^_^恭喜您,${chalk.underline.yellow(projectName)}项目部署成功了^_^\n`));
process.exit(0);
})
.catch((err) => {
console.log(chalk.red(' 文件解压失败', err));
process.exit(0);
});
}
// 执行Linux命令
function runCommand(command, webDir) {
return new Promise((resolve, reject) => {
ssh
.execCommand(command, { cwd: webDir })
.then((result) => {
resolve();
// if (result.stdout) {
// successLog(result.stdout);
// }
if (result.stderr) {
console.log(chalk.red(result.stderr));
process.exit(1);
}
})
.catch((err) => {
reject(err);
});
});
}
const config = require('./deploy.config.cjs');
startZip(config);